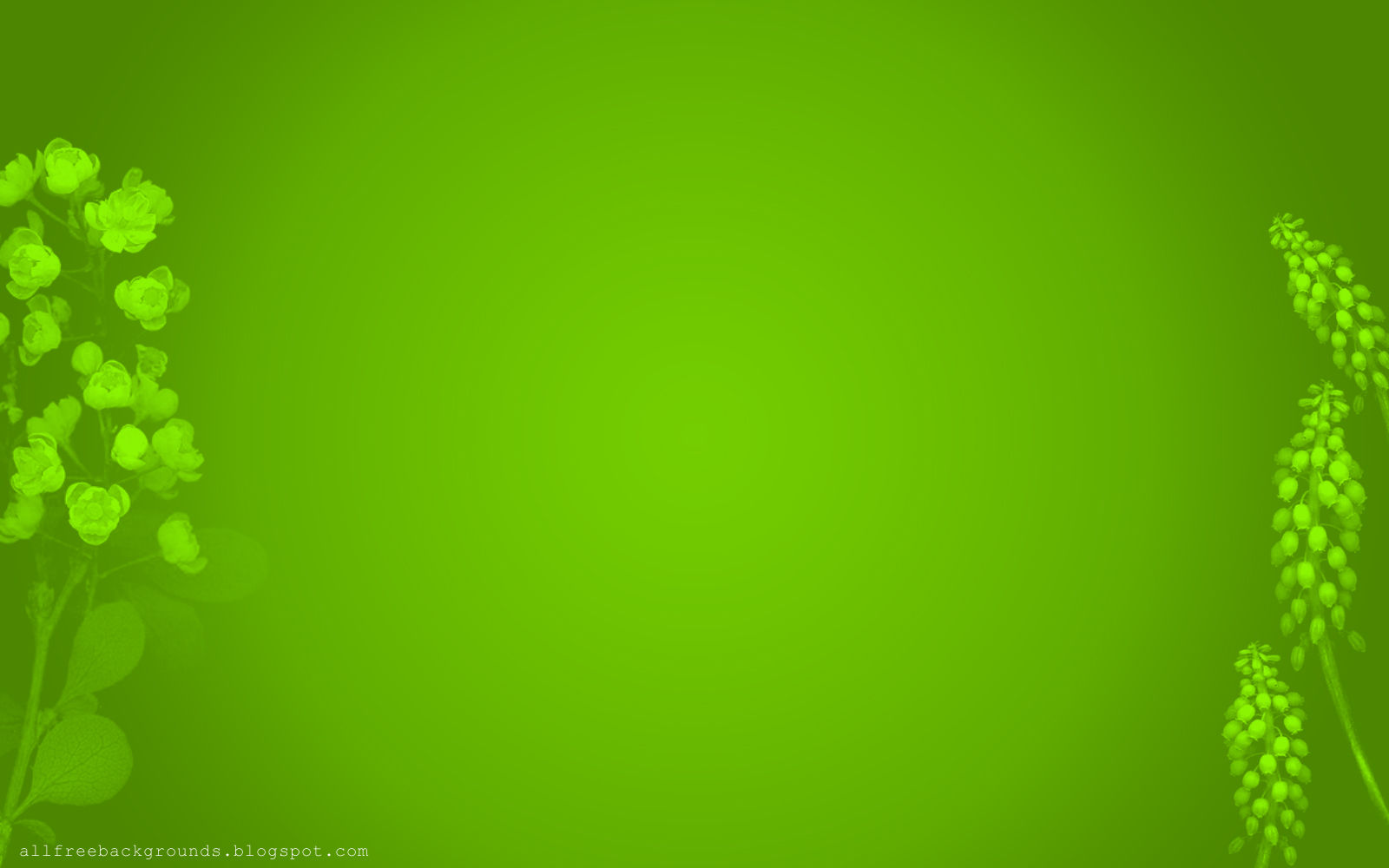
Software
-This is not the intended final code. Optimally this will use state machine (switch) coding, however right now this uses polling.
#include <DS3231.h>
// Init the DS3231 using the hardware interface
#include <Wire.h>
#include <Adafruit_SSD1306.h>
#include <Adafruit_GFX.h>
//#include <Adafruit_TFTLCD.h>
#include <Fonts/FreeSerif9pt7b.h>
DS3231 rtc(SDA, SCL);
// OLED display TWI address
#define OLED_ADDR 0x3C
int x = 14;
// reset pin not used on 4-pin OLED module
Adafruit_SSD1306 display(-1); // -1 = no reset pin
// set pin numbers:
int button = A1; // the number of the pushbutton pin
int button2 = A0;
// 128 x 32 pixel display
#if (SSD1306_LCDHEIGHT != 32)
#error("Height incorrect, please fix Adafruit_SSD1306.h!");
#endif
#include <dht.h>
dht DHT;
#define DHT11_PIN2 11
#define DHT11_PIN 10
int watercount = 0;
int lightcount = 0;
int chk;
int chk2;
int lightSensorpin = A7;
int lightSensorpin2 = A6;
int Relay = 12;
int Relay2= 13;
int analogValue = 0;
int analogValue2 = 0;
int motor = 2;
int motor2 = 3;
int digitalValue = 0;
int digitalValue2 = 0;
int watersensorValue =0;
int watersensorValue2 = 0;
void setup()
{
Serial.begin(9600);
pinMode(Relay, OUTPUT); //Set Pin12 as output
pinMode(Relay2, OUTPUT);
digitalWrite(Relay, HIGH); //HIGH IS OFF
digitalWrite(Relay2, HIGH);
pinMode(motor, OUTPUT);
pinMode(motor2, OUTPUT);
// initialize and clear display
display.begin(SSD1306_SWITCHCAPVCC, OLED_ADDR);
display.clearDisplay();
display.display();
// init the pushbutton pin as an input
pinMode(button, INPUT_PULLUP);
pinMode(button2, INPUT_PULLUP);
//display test w/ text
display.setTextSize(1);
display.setTextColor(WHITE,BLACK);
display.setCursor(0,0);
rtc.begin();
// The following lines can be uncommented to set the date and time
//rtc.setDOW(TUESDAY); // Set Day-of-Week to SUNDAY
//rtc.setTime(9, 18, 0); // Set the time to 12:00:00 (24hr format)
//rtc.setDate(5, 8, 2018); // Set the date to January 1st, 2014
}
void loop()
{
//Real Time Clock
// Send Day-of-Week
/*
Serial.print(rtc.getDOWStr());
Serial.print(" ");
// Send date
Serial.print(rtc.getDateStr());
Serial.print(" -- ");
// Send time
Serial.println(rtc.getTimeStr());
*/
//moisture and light sensors
analogValue = analogRead(lightSensorpin);
analogValue2 = 2.7*3.7*analogRead(lightSensorpin2);
watersensorValue = analogRead(A3);
watersensorValue2 = analogRead(A2);
/* Serial.print("Light = ");
Serial.println(analogValue);
Serial.print("Light2 = ");
Serial.println(analogValue2);
Serial.print("Moisture = ");
Serial.println(watersensorValue);
Serial.print("Moisture2 = ");
Serial.println(watersensorValue2);
*/
//Temperature/Humidity sensors
chk = DHT.read11(DHT11_PIN);
chk2 = DHT.read11(DHT11_PIN2);
/*Serial.print("Temperature = ");
Serial.println(DHT.temperature);
Serial.print("Temperature2 = ");
Serial.println(DHT.temperature);
Serial.print("Humidity = ");
Serial.println(DHT.humidity);
Serial.print("Humidity2 = ");
Serial.println(DHT.humidity);
*/
//OLED Display
// put your main code here, to run repeatedly:
display.clearDisplay();
// display.display();
display.setCursor(0,0);
//display.print(rtc.getDOWStr());
//display.print(" ");
// Send date
display.print(rtc.getDateStr());
display.print("-");
// Send time
display.println(rtc.getTimeStr());
display.print("Light = ");
display.print(analogValue);
display.print("/");
display.println(analogValue2);
display.print("Moisture = ");
display.print(watersensorValue);
display.print("/");
display.println(watersensorValue2);
display.print("Temp = ");
display.print(DHT.temperature);
display.print("/");
display.println(DHT.temperature);
//display.print("Humidity = ");
//display.print(DHT.humidity);
//display.print("Humidity2 = ");
//display.println(DHT.humidity);
display.display();
delay(2000);
if (watersensorValue < 700 /*&& rtc.getTimeStr() == 2 && watercount<5*/){ //has problem
//watercount++;
//lower the number more moist
digitalWrite(motor, LOW);
Serial.println(motor);}
else
{
digitalWrite(motor, HIGH); //Sets motor OFF
}
if (watersensorValue2 < 700 ){ //has problem would like to add && rtc.getTimeStr() == 05:00:00 //&& watercount < 6)
//watercount++;
digitalWrite(motor2, LOW);
Serial.println(motor2);}
else
{
digitalWrite(motor2, HIGH); //Sets motor OFF
}
if (analogValue > 950)// would like to add && 05:00:00 < (rtc.getTimeStr() < 05:00:30 && //lightcount < 6)
{ // higher the number darker it is
//lightcount++;
digitalWrite(Relay, LOW); //sets LEDs ON
}
else
{
digitalWrite(Relay,HIGH); //Sets LEDs OFF
}
if (analogValue2 > 880)// the point at which the state of LEDs change
{
digitalWrite(Relay2, LOW); //sets LEDs ON
}
else
{
digitalWrite(Relay2,HIGH); //Sets LEDs OFF
}
}